mirror of
https://github.com/restic/restic.git
synced 2024-12-22 15:57:07 +00:00
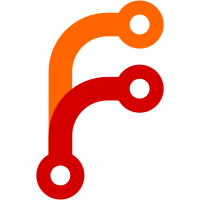
UnusedBlobs now directly reads the list of existing blobs from the repository index. This removes the need for the blobStatusExists flag, which in turn allows converting the blobRefs map into a BlobSet.
52 lines
931 B
Go
52 lines
931 B
Go
package checker
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/restic/restic/internal/restic"
|
|
)
|
|
|
|
// TestCheckRepo runs the checker on repo.
|
|
func TestCheckRepo(t testing.TB, repo restic.Repository) {
|
|
chkr := New(repo)
|
|
|
|
hints, errs := chkr.LoadIndex(context.TODO())
|
|
if len(errs) != 0 {
|
|
t.Fatalf("errors loading index: %v", errs)
|
|
}
|
|
|
|
if len(hints) != 0 {
|
|
t.Fatalf("errors loading index: %v", hints)
|
|
}
|
|
|
|
// packs
|
|
errChan := make(chan error)
|
|
go chkr.Packs(context.TODO(), errChan)
|
|
|
|
for err := range errChan {
|
|
t.Error(err)
|
|
}
|
|
|
|
// structure
|
|
errChan = make(chan error)
|
|
go chkr.Structure(context.TODO(), errChan)
|
|
|
|
for err := range errChan {
|
|
t.Error(err)
|
|
}
|
|
|
|
// unused blobs
|
|
blobs := chkr.UnusedBlobs(context.TODO())
|
|
if len(blobs) > 0 {
|
|
t.Errorf("unused blobs found: %v", blobs)
|
|
}
|
|
|
|
// read data
|
|
errChan = make(chan error)
|
|
go chkr.ReadData(context.TODO(), errChan)
|
|
|
|
for err := range errChan {
|
|
t.Error(err)
|
|
}
|
|
}
|